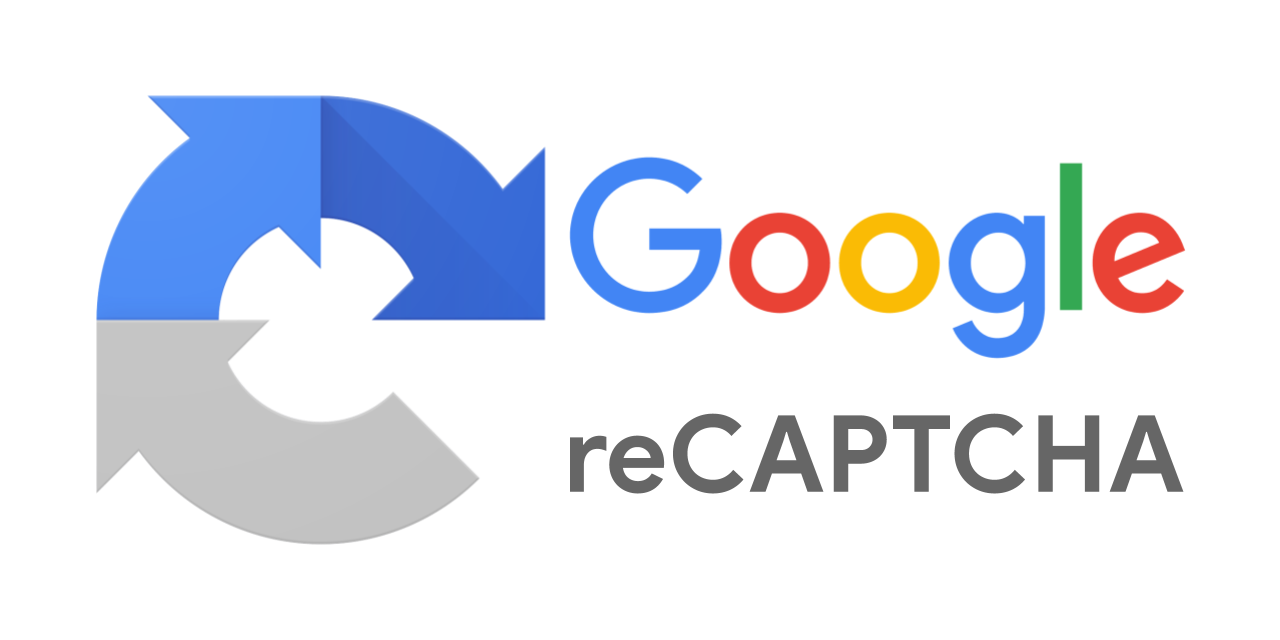
HTML code
to render the reCAPTCHA on the front-end.
index.html
<html> <head> <title>Google recapcha v3 demo</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> <script src="https://www.google.com/recaptcha/api.js?render=******YOUR-SITE-KEY******"></script> </head> <body> <h1>Google reCAPTHA Demo</h1> <form id="comment_form"> <input type="email" name="email" placeholder="Type your email" size="40"><br><br> <textarea name="comment" rows="8" cols="39"></textarea><br><br> <input type="submit" name="submit" value="Post comment"><br><br> </form> <script src="script.js"></script> </body> </html>
replace ******YOUR-SITE-KEY****** with your site key.
script.js
$('#comment_form').submit(function() { // we stoped it event.preventDefault(); var email = $('#email').val(); var comment = $("#comment").val(); // wait for recaptacha ready grecaptcha.ready(function() { // do request for recaptcha token // response is promise with passed token grecaptcha.execute('******YOUR-SITE-KEY******', {action: 'create_comment'}).then(function(token) { // add token to form $('#comment_form').prepend('<input type="hidden" name="g-recaptcha-response" value="' + token + '">'); $.post("form.php",{email: email, comment: comment, token: token}, function(result) { if(result.success) { alert('Thanks for posting comment.') } else { alert('You are spammer ! Get the @$%K out.') } }); }); }); });
replace ******YOUR-SITE-KEY****** with your site key.
server-side
On the server-side we are going to use PHP for now. So on the Form submit, we will check the POST variable.
form.php
<?php $email;$comment;$captcha; $email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL); $comment = filter_input(INPUT_POST, 'comment', FILTER_SANITIZE_STRING); $captcha = filter_input(INPUT_POST, 'token', FILTER_SANITIZE_STRING); if(!$captcha){ echo '<h2>Please check the captcha form.</h2>'; exit; } $secretKey = "******YOUR-SECRET-KEY******"; $ip = $_SERVER['REMOTE_ADDR']; // post request to server $url = 'https://www.google.com/recaptcha/api/siteverify'; $data = array('secret' => $secretKey, 'response' => $captcha, 'remoteip' => $ip); $options = array( 'http' => array( 'header' => "Content-type: application/x-www-form-urlencoded\r\n", 'method' => 'POST', 'content' => http_build_query($data) ) ); $context = stream_context_create($options); $response = file_get_contents($url, false, $context); $responseKeys = json_decode($response, true); header('Content-type: application/json'); if($responseKeys["success"] && $responseKeys["action"] === "create_comment" && $responseKeys["score"] >= 0.5) { echo json_encode(array('success' => 'true')); } else { echo json_encode(array('success' => 'false')); } ?>
replace ******YOUR-SECRET-KEY****** with your secret key.
Last Updated: Oct 17, 2024